generativepy.tween module
Categories: generativepy generative art
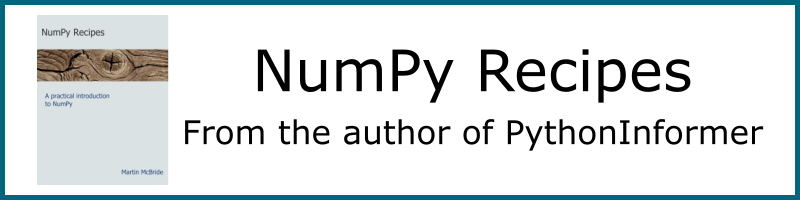
The tween module provides simple tweening functions, to help with animation when creating movies.
You would normally create a tween object with the same length as your movie. However, if the tween is shorter than the movie
You can build a tween object by adding sections that can:
- Maintain a constant value.
- Switch immediately to a new value at a particular time.
- Change linearly from one value to another over a certain length of time.
- Change in a non-linear way (easing) based on a supplied function.
After creating a tween object, you can access it using an index (like a tuple) to get the value of the variable for any particular frame.
There are 2 types of tween object:
Tween
tweens a single value.TweenVector
tweens a vector value of any dimension. It works on an element by element basis, for example if you tween from (x0, y0, z0) to (x1, y1, z1), the intermediate x values will vary linearly between x0 and x1, similar for y and z.
Times and frames
Tweens are mainly used for creating animation (image sequences, movies, or animated GIFs). Tween events are specified using times in seconds, because that is usually easier when designing an animation.
Tween values are read using a frame count, because you will normally need the tween value for a particular frame.
The Tween
module has a global frame rate, set by calling set_frame_rate
. This controls the conversion of time to frames.
Example
Here is an example that can be found on github as simpletween.py:
set_frame_rate(4)
# Create a tween. Times are in seconds (1 sec = 4 frames)
tween = Tween(3.0).wait(2).to(12.0, 5).wait(6.5).set(7.0).wait(7)
# You can use len() to fund the length of the tween in frames
print('Length = ', len(tween))
# You can access the value for a particular frame using indexing []
# or the get() function.
print('tween[6] (1.5 seconds) = ', tween[6])
print('tween[12] (3 seconds) = ', tween.get(12))
print('tween[80] (20 seconds) = ', tween.get(80))
# The tween can be accessed as a sequence. Here we use a list
# comprehension to convert the values to strings that print the
# entire sequence
strings = [str(x) for x in tween]
print(', '.join(strings))
We use set_frame_rate
to set a frame rate of 4 frames per second. You would normally use a higher rate (24 or more) for a good quality video, but for this example we use a low rate to reduce the amount of data.
Here we create a Tween
like this:
Tween(3.0)
creates an empty tween with an initial value of 3.0.wait(2)
maintains the current value (3.0) until 2 seconds in the video (ie frame 8).to(12.0, 5)
makes the value increase from 3.0 to 12.0 during the time from 2 seconds to 5 seconds (frame 9 to 20).wait(6.5)
maintains the current value (12.0) until 6.5 seconds in the video (ie frame 26).set(7.0)
sets the current value to 7.0. This gives a step change.wait(7)
maintains the current value (7.0) until 7 seconds in the video (ie frames 27, 28).
As the example shows, the tween acts like a sequence. We can use len
and indexing with []
ot get
. The index is the frame number, not the time. So when we access tween[6]
, we get the value for frame 6, which is 1.5 seconds into the video.
Notice that we can read tween[80]
even though the sequence is only 7 seconds (28 frames) long. Reaing past the end of the tween automatically returns the last value, which is 7.0 in this case.
In the last part of the example we convert the tween to a list of strings, and print it. The result is:
3.0, 3.0, 3.0, 3.0, 3.0, 3.0, 3.0, 3.0, 3.75, 4.5, 5.25, 6.0, 6.75, 7.5, 8.25, 9.0, 9.75, 10.5, 11.25, 12.0, 12.0, 12.0, 12.0, 12.0, 12.0, 12.0, 7.0, 7.0
This breaks down as:
Tween(3.0)
sets current value to 3.0wait(2)
adds 3.0, 3.0, 3.0, 3.0, 3.0, 3.0, 3.0, 3.0to(12.0, 5)
adds 3.75, 4.5, 5.25, 6.0, 6.75, 7.5, 8.25, 9.0, 9.75, 10.5, 11.25, 12.0wait(6.5)
adds 12.0, 12.0, 12.0, 12.0, 12.0, 12.0set(7.0)
sets the current value to 7.0. This gives a step change.wait(7)
adds 7.0, 7.0
Tween
Tween provides a constructor plus the methods:
wait
wait_d
set
to
to_d
get
Tween constructor
Creates an empty tween.
Tween(value=0)
Parameter | Type | Description |
---|---|---|
value | number | Initial current value. |
Tween.wait
Adds extra frames with the current value.
wait(time)
Parameter | Type | Description |
---|---|---|
time | float | Time to add |
Tween.wait_d
Adds extra frames with the current value.
wait_d(delta_time)
Parameter | Type | Description |
---|---|---|
delta_time | float | Time difference to add |
Similar to wait
but uses a delta time instead of absolute time. It is a convenience function to avoid having to calculate time deltas
Tween.set
Sets the current value.
set(value)
Parameter | Type | Description |
---|---|---|
value | number | New value. |
Tween.to
Adds extra frames that interpolate between the current value and a new value.
to(value, time)
Parameter | Type | Description |
---|---|---|
value | number | New value. |
time | float | Time to add |
Adds frames. The first frame will be set to the current values, the last frame will be set to to value
. The frames in between will be set to intermediate value using linear interpolation.
On exit, the new current value will be value
.
Tween.to_d
Adds extra frames that interpolate between the current value and a new value.
to_d(value, delta_time)
Parameter | Type | Description |
---|---|---|
value | number | New value. |
delta_time | float | Time difference to add |
Similar to to
but uses a delta time instead of absolute time. It is a convenience function to avoid having to calculate time deltas
Tween.ease
Adds extra frames that interpolate between the current value and a new value, according to an easing function.
ease(value, time, ease_function)
Parameter | Type | Description |
---|---|---|
value | number | New value. |
time | float | Time to add |
ease_function | function | Mapping function |
Adds frames. The frames will interpolate between the current value and the new value according to the ease_function
.
The ease_function
is a function that takes an input value in the range 0.0 to 1.0, and returns a value that varies from 0.0 to 1.0 by not usually in a linear way. The output value can also stray outside the range 0.0 to 1.0.
On exit, the new current value will be the result of the ease_function
applied to value
.
Tween.ease_d
Adds extra frames that interpolate between the current value and a new value, according to an easing function.
ease_d(value, time, ease_function)
Parameter | Type | Description |
---|---|---|
value | number | New value. |
time | float | Time to add |
ease_function | function | Mapping function |
Similar to ease
but uses a delta time instead of absolute time. It is a convenience function to avoid having to calculate time deltas
Tween.get
Gets the value at a specific frame.
get(frame)
Parameter | Type | Description |
---|---|---|
frame | int | Frame to get |
Gets the value of the frame with index frame
, which will be a number value.
TweenVector
TweenVector
is similar to Tween
but works with vector quantities.
TweenVector
is a subclass of Tween
. It inherits wait
, and get
from Tween
.
TweenVector constructor
Creates an empty tween.
TweenVector(value=(0, 0))
Parameter | Type | Description |
---|---|---|
value | number sequence | Initial current value. |
The value should be a list or tuple of length 2 or greater.
The dimension of the initial value determines the dimension of the tween. Every other value used (in set
or to
) must have the same dimension (eg if you start with a value of 3 elements, every value must have 3 elements).
TweenVector.set
Sets the current value.
set(value)
Parameter | Type | Description |
---|---|---|
value | number sequence | New value. |
The value
sequence must have the same number of elements as the initial value supplied in the constructor.
TweenVector.to
Adds extra frames that interpolate between the current value and a new value.
to(value, time)
Parameter | Type | Description |
---|---|---|
value | number sequence | New value. |
time | float | Time to add |
Adds frames. The first frame will be set to the current values, the last frame will be set to value
. The frames in between will be set to intermediate value using linear interpolation of each element of the value.
On exit, the new current value will be value
.
TweenVector.to_d
Adds extra frames that interpolate between the current value and a new value.
to_d(value, delta_time)
Parameter | Type | Description |
---|---|---|
value | number sequence | New value. |
delta_time | float | Time difference to add |
Similar to to
but uses a delta time instead of absolute time. It is a convenience function to avoid having to calculate time deltas
TweenVector.ease
Adds extra frames that interpolate between the current value and a new value, according to an easing function.
ease(value, time, ease_function)
Parameter | Type | Description |
---|---|---|
value | number sequence | New value. |
time | float | Time to add |
ease_function | function | Mapping function |
Adds frames. The frames in between will be set to intermediate values according to the ease_function
, which is applied to each element of the value.
The ease_function
is a function that takes an input value in the range 0.0 to 1.0, and returns a value that varies from 0.0 to 1.0 by not usually in a linear way. The output value can also stray outside the range 0.0 to 1.0.
On exit, the new current value will be the result of the ease_function
applied to value
.
TweenVector.ease_d
Adds extra frames that interpolate between the current value and a new value, according to an easing function.
ease_d(value, time, ease_function)
Parameter | Type | Description |
---|---|---|
value | number sequence | New value. |
time | float | Time to add |
ease_function | function | Mapping function |
Similar to ease
but uses a delta time instead of absolute time. It is a convenience function to avoid having to calculate time deltas
See also
- generativepy.analytics module
- generativepy.bitmap module
- generativepy.color module
- generativepy.drawing module
- generativepy.drawing3d module
- generativepy.formulas module
- generativepy.geometry module
- generativepy.geometry3d module
- generativepy.gif module
- generativepy.graph module
- generativepy.math module
- generativepy.movie module
- generativepy.nparray module
- generativepy.shape2d module
- generativepy.table module
- generativepy.utils module
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest