Tuples
Categories: python language beginning python
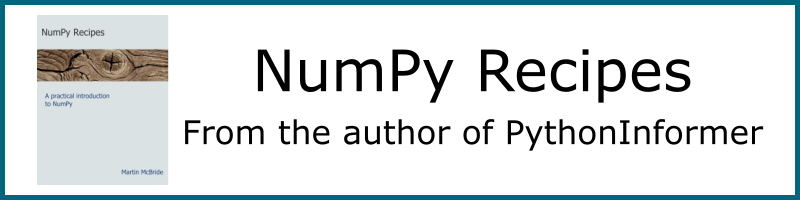
Introduction
In this lesson we will look at how to use tuples in Python:
- What is a tuple?
- Creating tuples
- Accessing elements
- Packing and unpacking tuples
- Tuple operations
What is a tuple?
Python tuples are very similar to Python lists.
They have a neater notation, which means they often get used for "quick" or temporary lists. Also, unlike a list, once you have created a tuple, you cannot change it.
An item which cannot be changed once it has been created is called immutable. Immutable objects are useful if you want to be sure that their value will not be changed accidentally.
You should read and understand the lists topic before you learn about tuples.
Creating a tuple
You can create a tuple using round brackets ()
. A tuple can contain any type of data, for example, numbers or strings. A tuple can contain a mixture of different types of data. Exactly like a list.
a = (1, 2, 3)
b = ('red', 'yellow', 'green', 'blue')
c = (4, 'pair', 'dozen', 15)
Creating an empty tuple
You can create an empty tuple as you might expect:
d = ()
Creating a tuple with just one element is a little bit tricky. You can't do this:
e = (3) #Wrong!
The problem is that this code will simply set e
equal to integer 3. To see the problem, look at this code:
x = 1 + (3)
The brackets around the (3)
aren't necessary, but you are allowed to put them in if you want. Python will evaluate the expression correctly (it will result in the value 4, of course). You wouldn't want Python to randomly create a tuple.
Instead you should do this:
e = (3,)
The extra comma tells Python that you really do want a tuple with one element.
Accessing elements
If you want to access individual elements, it is exactly the same as for a list:
a = (1, 2, 3)
x = a[2]
This reads element index 2 from the tuple and puts its value into variable x.
Note that you cannot set elements in a tuple. Remember, once a tuple is created you can't change it:
b = ('red', 'yellow', 'green', 'blue')
b[1] = 'orange' #Gives an error!
Packing and unpacking tuples
Packing is one of the things which sets tuples apart from lists.
Packing a tuple
Packing is a simple syntax which lets you create tuples "on the fly" without using the normal notation:
a = 1, 2, 3
This creates a tuple of 3 values and assigned it to a. Comparing this to the "normal" way:
a = (1, 2, 3)
OK, maybe it doesn't seem like much, but read on.
Unpacking a tuple
You can also go the other way, and unpack the values from a tuple into separate variables:
a = 1, 2, 3
x, y, z = a
After running this code, we will have x
set to 1, y
set to 2 and z
set to 3. The value of the tuple a
is unpacked into the 3 variables x
, y
and z
. Note that the number of variables has to exactly match the number of elements in the tuple, or there will be an error.
Real-life examples
Here is where it gets interesting. In the code above, the variable a is just used as a temporary store for the tuple. Why not leave out the middle-man and do this:
x, y, z = 1, 2, 3
After running this code, as before, we will have x
set to 1, y
set to 2 and z
set to 3.
Now it would be easy to look at that code and say, sure, Python has a special syntax for setting up multiple variables. But that isn't what is really happening. First the values are packed into a tuple:
Next, the tuple is assigned to the left-hand side of the = sign:
Finally, the tuple is unpacked into the variables:
Here is another example:
a = 5
b = 7
b, a = a, b
So what does that do? At first, you might scratch your head and wonder what on earth is going on.
But in fact, it is quite simple. First, a
and b
get packed into a tuple. Then the tuple gets unpacked again, but this time into variables b
then a
. So the values get swapped!
For a final example, let's see how tuple packing can be used with functions. A function is a bit of code that you can call to calculate a value. It can only return a single value. In this case, it is today's date (as a string):
def getDate():
#Get the date from the system in some way
return(date)
We can call it like this:
s = getDate()
print(s)
Now suppose we wanted to create a function that returns the current screen position of the mouse cursor. Clearly, this requires two values, the (x, y) position. Here is a neat way to do it:
def getMousePos():
#Get the mouse position the system in some way
return x, y
Do you recognise this? It is the tuple packing notation. x and y are packed into a tuple, which is a single value that can be returned by the function.
When you call the function, you can do the same thing in reverse:
u, v = getMousePos()
print(u, v)
Here you are unpacking the two values into variables u and v. It might look as if Python has some special syntax for passing back multiple values, but it doesn't … it is just tuples.
Tuple operations
Tuples use the same functions and constructs as lists, except of course you cannot do anything to modify a tuple.
Tuple slices
You can uses slices with tuples:
a = ['a', 'b', 'c', 'd', 'e']
y = a[1:4] #slice: ('b', 'c', 'd'), an list of elements 1, 2, and 3
If you take a slice of a tuple, you get a new tuple (and of course, if you take a slice of a list, you get a new list).
You cannot delete a slice of a tuple, because that would change the tuple itself, which isn't allowed - tuples are immutable.
Adding elements to a tuple
You cannot use append()
, insert()
or extend()
to add extra elements to a tuple, because that would be changing the tuple.
You can add two tuples, because that just creates a new tuple (it doesn't change a
or b
):
a = (1, 2, 3)
b = (10, 20, 30)
c = a + b
print c #(1, 2, 3, 10, 20, 30)
You can do something a bit like the insert()
function using slices:
a = (10, 20, 30, 40, 50)
x = a[:2] + ['apple', 'pear'] + a[2:] #x = (10, 20, 'apple', 'pear', 30, 40, 50)
Once again, this creates a new tuple, rather than modifying the existing tuple.
Finding elements
You can use the count()
and index()
functions to find elements in a tuple. You can use the in
and not in
operators to check for membership. The len()
function also works with tuples.
Removing elements
You cannot use remove()
, del
or clear()
on a tuple, for the obvious reason, again, that you cannot change a tuple. You can create a new tuple, with some of the elements removed.
Looping
The list looping techniques work perfectly well with tuples:
t = ('red', 'yellow', 'green', 'blue')
for x in t
print(x)
In case of emergency…
If you really must use list functions with a tuple, there is a function list() which will make a list out of anything it can, including a tuple:
t = ('red', 'yellow', 'green', 'blue')
k = list(t)
k.sort()
print(k)
Even in this case, you are not actually changing the tuple, only the list copy of it.
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest