Creating a simple pygame window
Categories: pygame
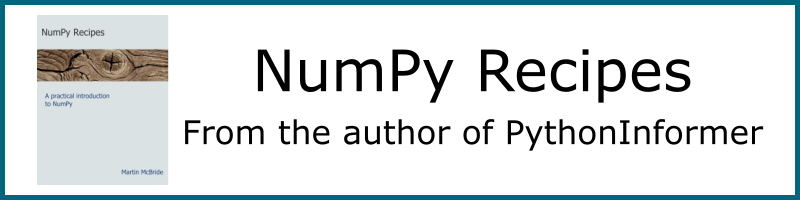
In this section we will create the most basic pygame program possible. It isn't even a game, it just displays an empty window, and waits for you to close it.
What is the point? Well it introduces the basic form that all pygame programs take, without any extra clutter or complexity. Pretty much every game you create will start off something like this.
Code structure
The basic structure of a pygame game is usually something like this:
initialise_the_game()
create_the_main_window()
# Main game loop
running = True
while running:
check_user_input()
update_game_state()
if finished:
running = false
close_pygame()
We start by initialising the pygame module, and anything else that our game needs - characters, props, sounds, number of lives and so on.
We then create the main window. Every game needs a window, just like any other UI program.
Next, we enter the main game loop. This loop will run forever, or until the user has had enough of it. It uses a boolean called running
that controls the loop. If we set running
to false anywhere in the loop, the game will end.
Inside the loop we basically do two main things:
- Check of any user input, such as pressing a key, or moving the mouse or joystick.
- Update the game state - the main part of the game where we move objects around, check for collisions, make sounds, and points to the score and everything else.
Minimal game
In this section we will be implementing a cut down version of the code above, that does this:
initialise_pygame()
create_the_main_window()
# Main game loop
running = True
while running:
check_if_user_closes_window()
if finished:
running = false
There are no characters, props, sounds or anything else, for the time being. We will just display an empty window, and wait for the user to close the window using the close button on the title bar.
The code
Here is the code:
import pygame as pg
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# Initialise pygame
pg.init()
screen = pg.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
# Main loop, run until window closed
running = True
while running:
# Check events
for event in pg.event.get():
if event.type == pg.QUIT:
running = False
# close pygame
pg.quit()
Here is the result:
Here it is step by step. First some imports and definitions:
import pygame as pg
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
We import the main pygame module. We import it as pg
, which just means that everywhere in our code where we use pygame, we can call it pg
. It is just a bit shorter, and since we are using pygame all over the place, there isn't much chance we will forget what pg
means.
We also define the screen width and height in pixels.
Next we initialise pygame. That just lets pygame do anything it needs to before we start using it:
# Initialise pygame
pg.init()
The next line creates the window. We pass in the window size:
screen = pg.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
Now here is the main loop:
# Main loop, run until window closed
running = True
while running:
# Check events
for event in pg.event.get():
if event.type == pg.QUIT:
running = False
The main thing this loop does is to call pg.event.get()
. This detects any "events" that might have happened. These are things like key presses and mouse clicks.
pg.event.get()
actually returns a list of events. That is because it is possible that more than one event can happen at the same time - for example you could move the mouse and press the space key at the same time. So the get
method returns a list of all the events, and our code loops over the events looking for a QUIT
event. We ignore any other events.
The list will be empty if no event has happened.
Finally, we quit pygame. This gives pygame a chance to tidy up after itself:
# close pygame
pg.quit()
Running the code
When you run the code, you should just see a small window with a black background. If you close the window (by clicking the X button in the title bar) the window will close and the program will end.
The source code is available on github as simplewindow.py.
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest