Cryptography getting started
Categories: cryptography
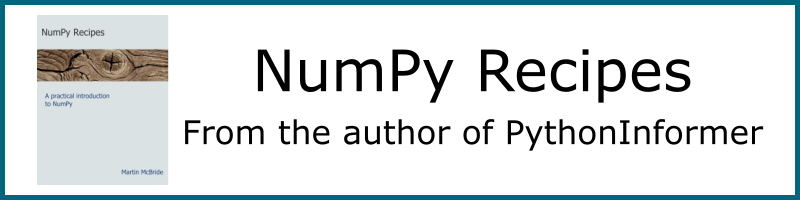
This article is part of a series on the Python cryptography library.
The cryptography module can be installed using pip, like this:
pip install cryptography
If you use Anaconda, you can also install cryptography in the usual way with conda.
Building the module yourself
Both pip and conda download a pre-built executable from a repository. There is, at least theoretically, a possibility of an attacker hacking the repository and replacing the original executable with their own doctored version, that might contain back doors.
If your application requires a high degree of security, you should consider building the library yourself. Instructions for doing this are included on the official cryptography module website at cryptography.io.
Checking the module
You can check that the module is installed correctly using this simple program that creates a key then encodes and decodes a message:
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
# Create a Fernet instance with the key
cipher = Fernet(key)
# Encrypt a message
message = "Message to be encrypted".encode('utf-8')
token = cipher.encrypt(message)
print(token.hex())
# Decrypt the cipertext
decoded = cipher.decrypt(token)
print(decoded)
The line print(token.hex())
should print the encrypted message, something like:
674141414141426555704e5a6e423947
333562524c504b333751745a47576432
3266305a6a33356c3645304838425446
316e5a58627850634741425f30545576
6b637956526e30664d75523337325261
776a543375785a677a64434353527142
61516e7446766f326f6d4a6c4d313363
84449776f4a673d
The encrypted data will be different every time, because it contains random elements.
The line print(decoded)
prints the result of decrypting the token
. It should be:
b'Message to be encrypted'
We will look at this code in more detail in the Fernet encryption section.
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest